Ok. So in this tutorial I'll start with a very short intro to what OOP is, and then the real tutorial will be using OOP to program a guy moving. This may be the first tutorial in the series.
What is OOP?
Object Oriented Programming. That's what it is. Flash basically already has this built in, you use OOP when you use an mc's properties, or a variables properties. That's what OOP is, programming with Objects and properties. I could ramble on and on about what it is. For more info go to Kirupa.com they have a very extensive collection of OOP tutorials that take forever to go through and don't get to actually programming it enough.
LETS GO!!!
Okay. So we need to make a char move. First place an mc on the stage, instance name of hero.
Now lets make the Construtor of the Player.
Player = function(graphic)
{
this.graphic = graphic; //Define our graphic
this.graphic.controller = this; //Define our graphic's controller
this.graphic.onEnterFrame = function() //Use the graphic to update Player every frame
{
this.controller.InGameFunctions(); //Call our InGameFunctions function.
}
}
Now we make our Move function()
Player.prototype.Move = function()
{
if(Key.isDown(Key.UP))
this.graphic._y -= 10;
if(Key.isDown(Key.DOWN))
this.graphic._y += 10;
if(Key.isDown(Key.LEFT))
this.graphic._x -= 10;
if(Key.isDown(Key.RIGHT))
this.graphic._x += 10;
}
Player.prototype.Move means that we want to add a function to the Player class.
We can use this.graphic because that means whatever the Player is.graphic basically.
Player.prototype.InGameFunctions = function()
{
this.Move(); //this.Move() because its a Player function not a global one.
}
Test it... WHAT?? It's not moving? Oh, that's right, we havn't made an instance of Player yet.
Hero = new Player(hero);
hero is the graphic's instance name.
Any questions? comment.
Apr 22, 2007
Subscribe to:
Post Comments (Atom)
FlashGrounds
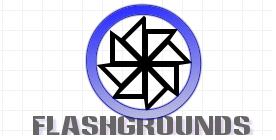
Kevin Stubbs, Ivan Alvarez Malo
1 comment:
Hi, Are you using an external actionscript file? - Great tute if i can figure it out.
Post a Comment