Ok. So in this tutorial I'll start with a very short intro to what OOP is, and then the real tutorial will be using OOP to program a guy moving. This may be the first tutorial in the series.
What is OOP?
Object Oriented Programming. That's what it is. Flash basically already has this built in, you use OOP when you use an mc's properties, or a variables properties. That's what OOP is, programming with Objects and properties. I could ramble on and on about what it is. For more info go to Kirupa.com they have a very extensive collection of OOP tutorials that take forever to go through and don't get to actually programming it enough.
LETS GO!!!
Okay. So we need to make a char move. First place an mc on the stage, instance name of hero.
Now lets make the Construtor of the Player.
Player = function(graphic)
{
this.graphic = graphic; //Define our graphic
this.graphic.controller = this; //Define our graphic's controller
this.graphic.onEnterFrame = function() //Use the graphic to update Player every frame
{
this.controller.InGameFunctions(); //Call our InGameFunctions function.
}
}
Now we make our Move function()
Player.prototype.Move = function()
{
if(Key.isDown(Key.UP))
this.graphic._y -= 10;
if(Key.isDown(Key.DOWN))
this.graphic._y += 10;
if(Key.isDown(Key.LEFT))
this.graphic._x -= 10;
if(Key.isDown(Key.RIGHT))
this.graphic._x += 10;
}
Player.prototype.Move means that we want to add a function to the Player class.
We can use this.graphic because that means whatever the Player is.graphic basically.
Player.prototype.InGameFunctions = function()
{
this.Move(); //this.Move() because its a Player function not a global one.
}
Test it... WHAT?? It's not moving? Oh, that's right, we havn't made an instance of Player yet.
Hero = new Player(hero);
hero is the graphic's instance name.
Any questions? comment.
Apr 22, 2007
Apr 11, 2007
Make Setting up Input Easier.
Alright. Well here's a short tut about how to do keyboard input without having to remember or lookup ASCII codes. What are ASCII codes? ASCII codes are the numbers that were in Ivan's keyboard input tutorial for the Key.isDown(37) for example. 37 is an ASCII number that stands for the left arrow key. Like in this example:
onEnterFrame = function()
{
if(Key.isDown(37))
{
//left arrow has been pressed
trace("left arrow has been pressed!');
}
}
run it, when you press Left arrow key it should output that.
Lets say you're creating a game that takes keyboard input WASD. It would be so easy if we defined the ASCII codes for each key and then not use "magic" numbers.
Key.isDown(W) instead of Key.isDown(87) . Makes programming a lot easier.
So let's define some variables.
W = 87;
A = 71;
D = 74;
S = 83;
UP = Key.UP;
DOWN = Key.DOWN;
LEFT = Key.LEFT;
RIGHT = Key.RIGHT;
And use that. In fact, how about we create a .as script that defines all of these codes.
Just create a new .as file and put that code into there. Now put that as file in the same folder as your game. Then in your game put #include "INPUT.as" without a ';' . INPUT.as is the file name that holds the key codes. Now if you wanted to do some keyboard input you'd do something like Key.isDown(W) and then it has the key code.
onEnterFrame = function()
{
if(Key.isDown(37))
{
//left arrow has been pressed
trace("left arrow has been pressed!');
}
}
run it, when you press Left arrow key it should output that.
Lets say you're creating a game that takes keyboard input WASD. It would be so easy if we defined the ASCII codes for each key and then not use "magic" numbers.
Key.isDown(W) instead of Key.isDown(87) . Makes programming a lot easier.
So let's define some variables.
W = 87;
A = 71;
D = 74;
S = 83;
UP = Key.UP;
DOWN = Key.DOWN;
LEFT = Key.LEFT;
RIGHT = Key.RIGHT;
And use that. In fact, how about we create a .as script that defines all of these codes.
Just create a new .as file and put that code into there. Now put that as file in the same folder as your game. Then in your game put #include "INPUT.as" without a ';' . INPUT.as is the file name that holds the key codes. Now if you wanted to do some keyboard input you'd do something like Key.isDown(W) and then it has the key code.
Apr 10, 2007
Best Flash site on the Web
Come and visit : www.kongregate.com
Here you can have a great variety of flash games, excelent forums and you can upload your own games.
Simply Great...
Here you can have a great variety of flash games, excelent forums and you can upload your own games.
Simply Great...
Flash Tuts and more
Hi I'm the other owner of this blog, we want to help anyone that is starting to work in Flash or someone that is looking for something in special.
I live in Mexico so if you are hispanic feel free to ask for a translation, my mail is ialvarezmalo@gmail.com so any question, suggestions and more feel free to e-mail me....
I live in Mexico so if you are hispanic feel free to ask for a translation, my mail is ialvarezmalo@gmail.com so any question, suggestions and more feel free to e-mail me....
Hello! A Blog about Flash that'll actually be useful!
Hello! Me (Stubbs) and Ivan have created this blog to give you help on flash by tutorials we've made or something you've personally asked us about. We will also be giving updates and post mortems on any games we create nomatter how big or small.
That's all I've got to say. Ivan, take it away.
That's all I've got to say. Ivan, take it away.
Subscribe to:
Posts (Atom)
FlashGrounds
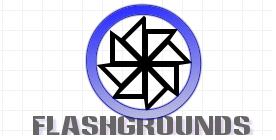
Kevin Stubbs, Ivan Alvarez Malo