Something I whipped up for myself in a few minutes.
This cuts down the code I need to play a sound by about 2 lines haha, but I think its pretty useful, check it out especially if sounds in Actionscript 3 aren't making sense for you. =)
FX.as -
package
{
import flash.display.MovieClip;
import flash.media.Sound;
import flash.media.SoundChannel;
public class FX extends MovieClip
{
var sound:Sound;
public function FX (type:Object)
{
sound = new type();
var fx:SoundChannel;
fx = sound.play();
}
}
}
Used like so:
var shot:FX = new FX(gunshot1);
gunshot1 is the class of the sound i want to use, right click your sound in the library (like gunshot.wav), and click linkage. Make its class be something like gunshot1, or mysfx or whatever.
Dec 21, 2008
Dec 20, 2008
Making the AS3.0 VCam Follow Your Player
This tutorial is directed to people who know a bit about flash, I won't be explaining everything in detail.
Download the VCam from this site if you don't already have it.
http://bryanheisey.com/blog/?p=1
Make a blank document, don't try this on a game you're working on right now, because if you mess something up then it could be a headache for you. Best to just learn it fast and then implement it afterwards.
So, add the vcam into your document. Go into the vcam's code (double click the vcam mc).
We need to do some editing to make this work right, find this line and delete it:
addEventListener(Event.ENTER_FRAME,camControl);
We are going to be calling the camControl function from our player after and before we move it, that way there should be no jitters.
Now make a player mc, I used a picture of Borat.
Before he moves, and after he moves, you want to update the camera. I found, for this example at least that if you also update it before the movement it takes the jitter away, if you don't do that then there's some jitter I'm not sure why.
The code is too long for a post, so look at the fla instead if you need an idea of how to do this.
ZIP FILE
Download the VCam from this site if you don't already have it.
http://bryanheisey.com/blog/?p=1
Make a blank document, don't try this on a game you're working on right now, because if you mess something up then it could be a headache for you. Best to just learn it fast and then implement it afterwards.
So, add the vcam into your document. Go into the vcam's code (double click the vcam mc).
We need to do some editing to make this work right, find this line and delete it:
addEventListener(Event.ENTER_FRAME,camControl);
We are going to be calling the camControl function from our player after and before we move it, that way there should be no jitters.
Now make a player mc, I used a picture of Borat.
Before he moves, and after he moves, you want to update the camera. I found, for this example at least that if you also update it before the movement it takes the jitter away, if you don't do that then there's some jitter I'm not sure why.
The code is too long for a post, so look at the fla instead if you need an idea of how to do this.
ZIP FILE
Embedding SWF's Into Your Blog
http://bookoftips.blogspot.com/2008/02/tips-on-how-to-embed-swf-flash-movies.html
Great, easy tutorial with code that actually works (unlike some of the other articles...)
Great, easy tutorial with code that actually works (unlike some of the other articles...)
Simple Buttons in AS3.0
It's basically like AS2 and 1, but instead of sticking the code onto the buttons themselves, we just have a seperate class that handles it. Using seperate files for your projects might seem a bit daunting at first coming straight from "no oop" (as2 and 1 still uses oop even without those seperate files) as2 and as1. But really its not. Now, in as3 there is no this.onRelease or this.onEnterFrame and what not. No, its all events now, which is easy, and probably much easier to pick through as well.
So we'll have the fla that holds the button and a dynamic text instance to show us what the 'game' is doing. It's just going to say "pressed" or "rolled over" or whatever. We will also have an extra .as file that will make everything work.
Starting:
Create these two blank files.Make a button named mybutton in the fla, and make a dynamic text instance named etxt. The document class (found in the properties tab) should be Game. It will say there is no definition available, but we are about to make the file so this message doesn't matter.
The structure of our .as file should look like this:
package
{
import flash.display.*; //For our button and movieclip
import flash.text.*; //For our dynamic text
import flash.events.*; //For our events..
public class Game extends MovieClip
{
}
}
You will eventually know how to do this by heart, and it will probably feel strange the first few times you type this out.We needed to import those 3 libraries to use those things.You could be specific and probably save some speed if you did something like import flash.display.Movieclip; import flash.display.SimpleButton instead of flash.display.*;But I don't think it really matters, this is only a small example of a button we don't need to be cautious about lag.When you import a library, like flash.display.*;The asteriks (*) tells the computer to just import everything under the flash.display library, this saves us from having to individually type each class we want to import.
Now we'll do the meat of the code. The bolded code is the new code.
package
{
import flash.display.*;
import flash.text.*;
import flash.events.*;
public class Game extends MovieClip
{
public function Game()
{
mybutton.addEventListener(MouseEvent.ROLL_OVER, RollOver);
mybutton.addEventListener(MouseEvent.ROLL_OUT, RollOut);
mybutton.addEventListener(MouseEvent.MOUSE_UP, Released);
mybutton.addEventListener(MouseEvent.MOUSE_DOWN, Pressed);
}
public function RollOver(event:MouseEvent)
{
etxt.text = "Rolled Over";
}
public function RollOut(event:MouseEvent)
{
etxt.text = "Rolled Out";
}
public function Released(event:MouseEvent)
{
etxt.text = "Released";
}
public function Pressed(event:MouseEvent)
{
etxt.text = "Pressed";
}
}
}
Our constructor is where we tell the computer what to do when these events occur. Let's take this line for example.mybutton.addEventListener(MouseEvent.ROLL_OVER, RollOver);We are saying, when mybutton has been rolled over, call the RollOver function that we define ourselves.It's actually very very simple.
And then we just have those 4 functions defined right below our constructor method, the only thing the functions do is change the text of that dynamic etxt to let us know what just happened.
That's it, hopefully you learned about buttons and some key ideas of Actionscript 3.0
ZIP FILE
It's basically like AS2 and 1, but instead of sticking the code onto the buttons themselves, we just have a seperate class that handles it. Using seperate files for your projects might seem a bit daunting at first coming straight from "no oop" (as2 and 1 still uses oop even without those seperate files) as2 and as1. But really its not. Now, in as3 there is no this.onRelease or this.onEnterFrame and what not. No, its all events now, which is easy, and probably much easier to pick through as well.
So we'll have the fla that holds the button and a dynamic text instance to show us what the 'game' is doing. It's just going to say "pressed" or "rolled over" or whatever. We will also have an extra .as file that will make everything work.
Starting:
Create these two blank files.Make a button named mybutton in the fla, and make a dynamic text instance named etxt. The document class (found in the properties tab) should be Game. It will say there is no definition available, but we are about to make the file so this message doesn't matter.
The structure of our .as file should look like this:
package
{
import flash.display.*; //For our button and movieclip
import flash.text.*; //For our dynamic text
import flash.events.*; //For our events..
public class Game extends MovieClip
{
}
}
You will eventually know how to do this by heart, and it will probably feel strange the first few times you type this out.We needed to import those 3 libraries to use those things.You could be specific and probably save some speed if you did something like import flash.display.Movieclip; import flash.display.SimpleButton instead of flash.display.*;But I don't think it really matters, this is only a small example of a button we don't need to be cautious about lag.When you import a library, like flash.display.*;The asteriks (*) tells the computer to just import everything under the flash.display library, this saves us from having to individually type each class we want to import.
Now we'll do the meat of the code. The bolded code is the new code.
package
{
import flash.display.*;
import flash.text.*;
import flash.events.*;
public class Game extends MovieClip
{
public function Game()
{
mybutton.addEventListener(MouseEvent.ROLL_OVER, RollOver);
mybutton.addEventListener(MouseEvent.ROLL_OUT, RollOut);
mybutton.addEventListener(MouseEvent.MOUSE_UP, Released);
mybutton.addEventListener(MouseEvent.MOUSE_DOWN, Pressed);
}
public function RollOver(event:MouseEvent)
{
etxt.text = "Rolled Over";
}
public function RollOut(event:MouseEvent)
{
etxt.text = "Rolled Out";
}
public function Released(event:MouseEvent)
{
etxt.text = "Released";
}
public function Pressed(event:MouseEvent)
{
etxt.text = "Pressed";
}
}
}
Our constructor is where we tell the computer what to do when these events occur. Let's take this line for example.mybutton.addEventListener(MouseEvent.ROLL_OVER, RollOver);We are saying, when mybutton has been rolled over, call the RollOver function that we define ourselves.It's actually very very simple.
And then we just have those 4 functions defined right below our constructor method, the only thing the functions do is change the text of that dynamic etxt to let us know what just happened.
That's it, hopefully you learned about buttons and some key ideas of Actionscript 3.0
ZIP FILE
Jul 8, 2008
Subscribe to:
Posts (Atom)
FlashGrounds
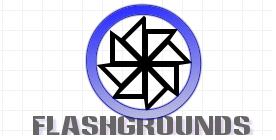
Kevin Stubbs, Ivan Alvarez Malo